There is a lot of lines of code in video games. There are teams of people who work on games now.
Here is some example of how code is implemented into programs to make it look like you are jumping. I got this example from the web site http://www.gamedev.net/reference/articles/article694.asp
this is pseudo-code that can be used for if you were having the character in the game jump straight up.
Joe.postion.y = 0; (Or wherever floor is)
Joe.velocity.y = 0;
Joe.acceleration = 10;
Joe.jumping = NO;
Loop
If (jump key is pressed)
{
Joe.velocity.y = 100;
Joe.jumping = YES;
}
if (Joe.jumping == YES) /* Move Joe if we are jumping */
{
Joe.velocity.y = Joe.velocity.y - Joe.acceleration
Joe.postion.y = Joe.postion.y + Joe.velocity.y
}
if (Joe.postion.y <= 0) /* Check to see if Joe has hit floor */
{
Joe.velocity.y = 0;
Joe.jumping = NO;
}
End Loop
this code will continue to run until joe hits the floor after the jump key is pressed.
The loop means that it will continue to run the statements on the inside of the loop until the loop fails in its test.
so the program will show this each times it loops.
Loop0 : Joe.velocity.y = 0 Joe.postion.y = 0
Loop1 : Joe.velocity.y = 100 Joe.postion.y = 100
Loop2 : Joe.velocity.y = 90 Joe.postion.y = 190
Loop3 : Joe.velocity.y = 80 Joe.postion.y = 270
Loop4 : Joe.velocity.y = 70 Joe.postion.y = 340
Loop5 : Joe.velocity.y = 60 Joe.postion.y = 400
Loop6 : Joe.velocity.y = 50 Joe.postion.y = 450
Loop7 : Joe.velocity.y = 40 Joe.postion.y = 490
Loop8 : Joe.velocity.y = 30 Joe.postion.y = 520
Loop9 : Joe.velocity.y = 20 Joe.postion.y = 540
Loop10: Joe.velocity.y = 10 Joe.postion.y = 550
Loop11: Joe.velocity.y = 0 Joe.postion.y = 550
Loop12: Joe.velocity.y = -10 Joe.postion.y = 540
Loop13: Joe.velocity.y = -20 Joe.postion.y = 520
Loop14: Joe.velocity.y = -30 Joe.postion.y = 490
Loop15: Joe.velocity.y = -40 Joe.postion.y = 450
Loop16: Joe.velocity.y = -50 Joe.postion.y = 400
Loop17: Joe.velocity.y = -60 Joe.postion.y = 340
Loop18: Joe.velocity.y = -70 Joe.postion.y = 270
Loop19: Joe.velocity.y = -80 Joe.postion.y = 190
Loop20: Joe.velocity.y = -90 Joe.postion.y = 100
Loop21: Joe.velocity.y =-100 Joe.postion.y = 0
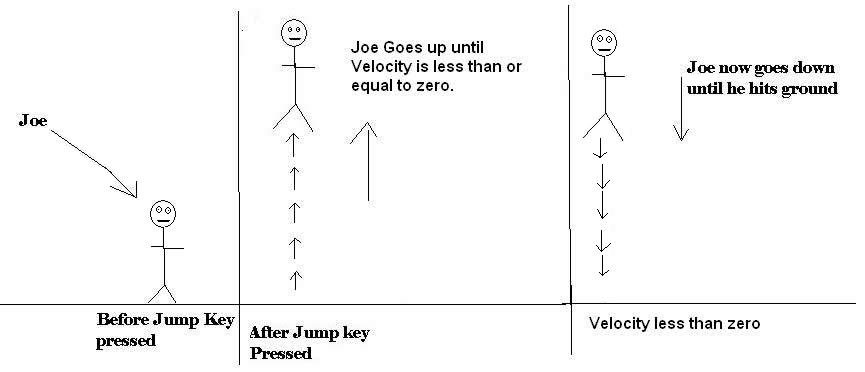
You notice once it starts after the jump key is pressed that he will go up to 100 units and continue to go up until his velocity equals zero. Then after velocity is less than zero he will go down until he hits the ground.
Rigid body physics is one the more complex equations that games have to go through. A deformable body can be modeled as a system of point masses connected by springs. The bodies can be curve masses(e.g., hair or rope), surface masses (e.g., cloth or the surface of a body of water), or volume masses (e.g., a gelatinous blob or a moving, viscous material). ( Eberly 165).
This means that most of the objects that are seen in games are just a certain amount of springs interconnected to create the object.
This image was made by me but i got the image from Eberly pg168
This is an image to represent the mass-spring system that would be 2D. These kind of dimensions are used for things like cloth.
This is just a really brief overview on what the physics in video games are used.to try to simulate real life situations. For a program to take ever point of something like a cloth and have it react to different things would be way too daunting on a program, so the mass-spring system is really good for it.